
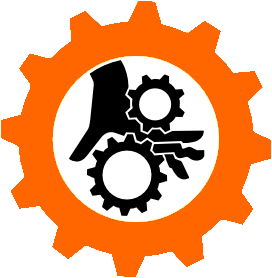
When
…I do not give Instagram or any entities associated with Facebook permission to use my pictures, information, messages or posts, both past and future. With this statement, I give notice to Instagram it is strictly forbidden to disclose, copy, distribute, or take any other action against me based on this profile and/or its contents.
Goes wrong
“They changed the only part of the article that I read! I’m gonna report this!!!”